1. Integration process
1.1 SDK
Unzip Framework.zip
Copy CoraoolLib.bundle to the application directory and introduce it into the project
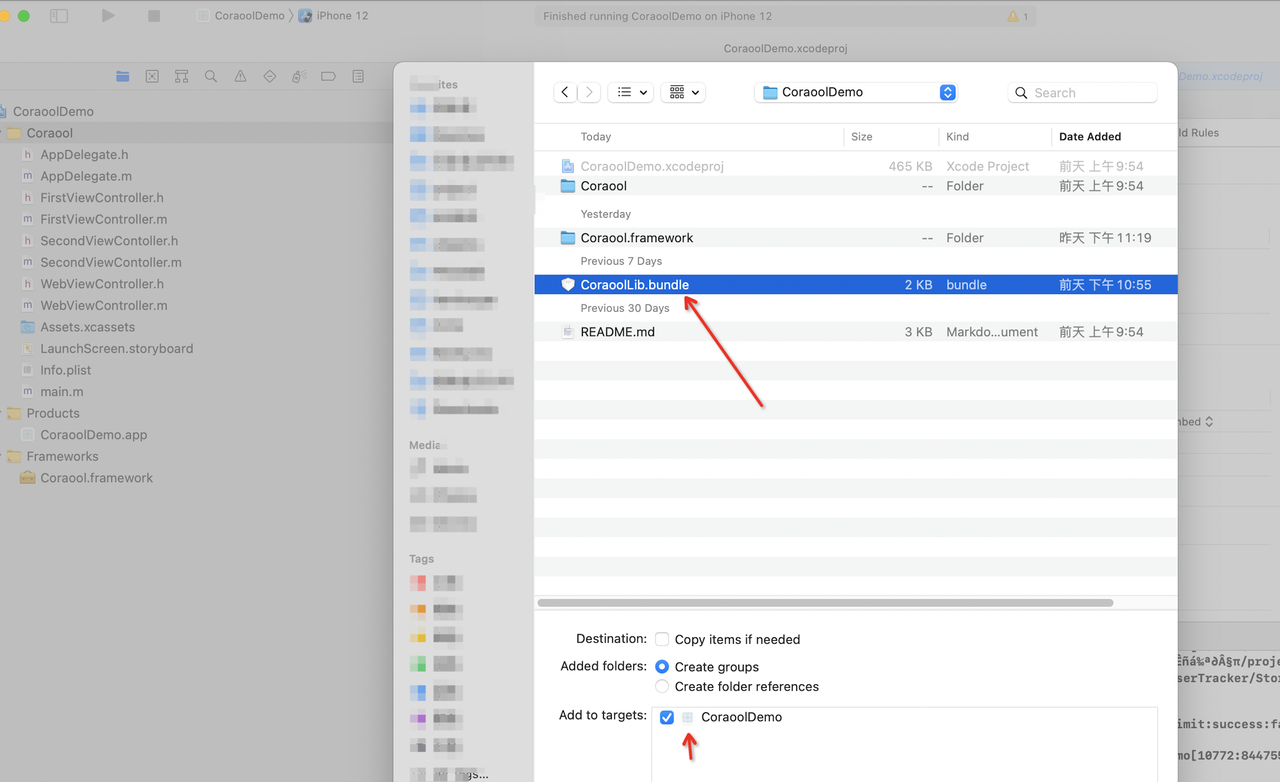
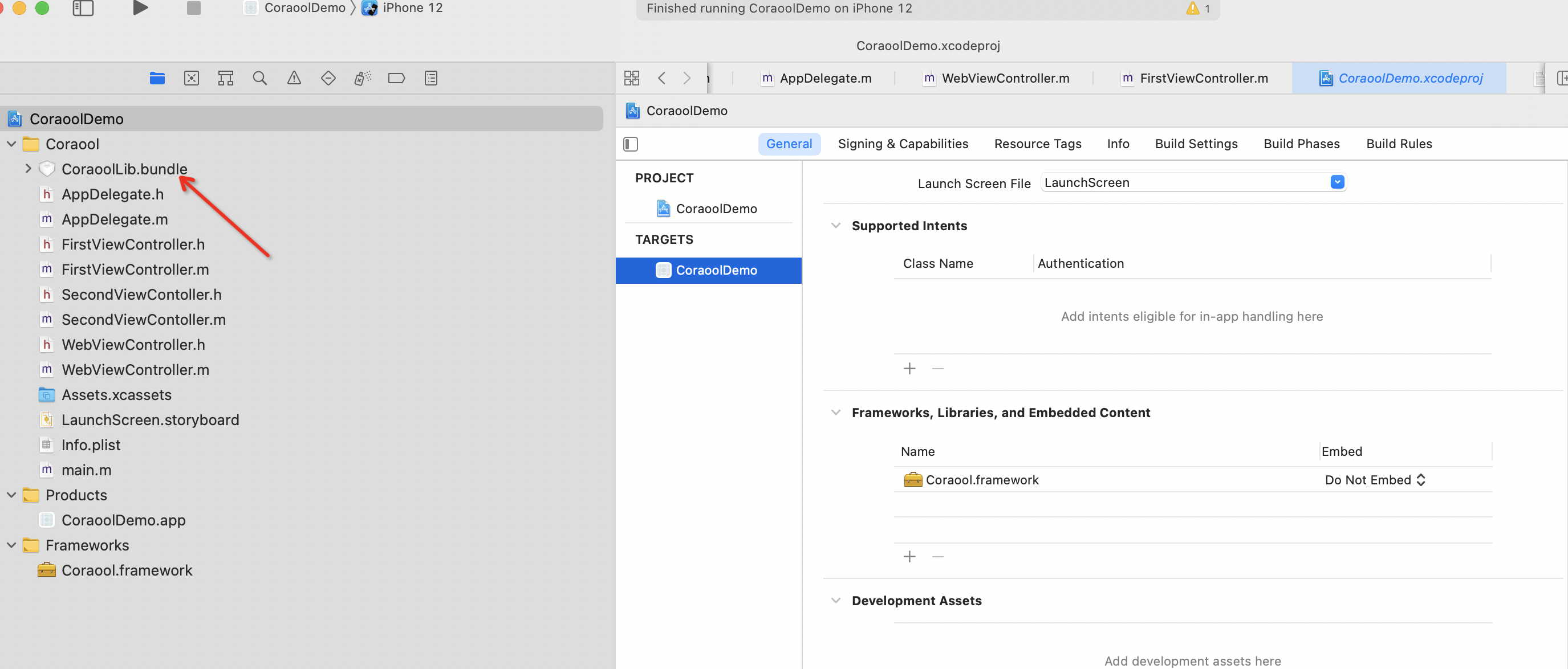
Copy the framework to the application directory, add it to the project, introduce the framework, and set Do not Embed
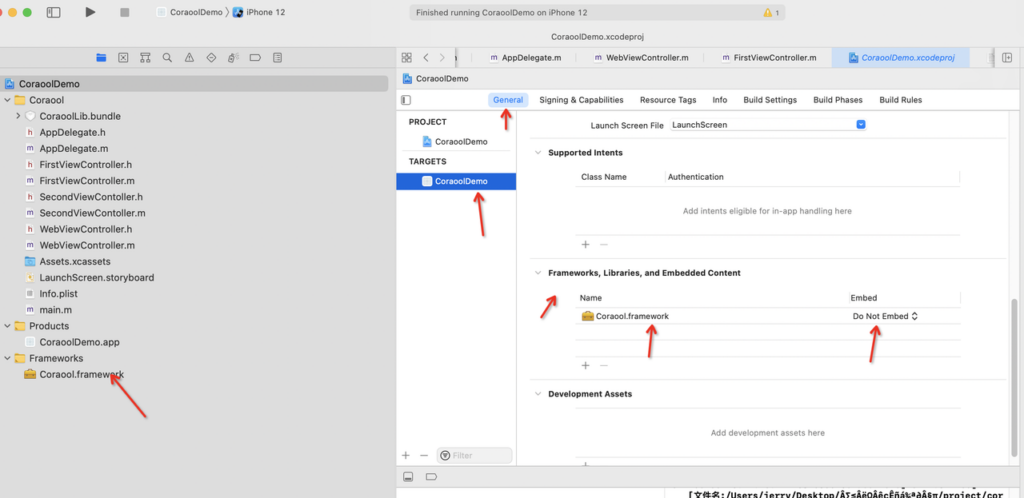
VersionDate | Release Notes | SDK |
---|---|---|
20240713 | 1. Increase AB ability (see Chapter 5 for details) The following 3 methods are mainly added: Get single experiment bucket getABTest Get a single experiment bucket object getABTestObject Get all experimental buckets getABTestListAll 2. Improve system stability and optimize SDK performance 3. Add internal dirty value detection | coraool-ios-sdk-1.0.9.zip |
20240619 | Improve system stability | coraool-ios-sdk-1.0.8.zip |
20240526 | Improve system stability | coraool-ios-sdk-1.0.7.zip |
20240518 | Improve system stability | coraool-ios-sdk-1.0.6.zip |
1.2 Configure SDK
1.2.1 Permission Grant
Permissions | Permission usage |
---|
1.3 Initialization steps
1.3.1 Apply for AK
Before accessing each app, you need to apply for AppId, AppKey, and AppSecret.
1.3.2 SDK initialization
The SDK is a single class, and the corresponding data can be directly used to set it.
/// 获取实例
+(CoraoolLib *)getInstance;
/// 【必须】设置app 的appId
///
/// 使用例子:
/// [[CoraoolLib getInstance] setAppId:@"c18"];
///
/// @param appId :设置app 的appId
-(void)setAppId:(NSString *)appId;
/// 【必须】设置appKey
///
/// 使用例子:
/// [[CoraoolLib getInstance] setAppKey:@"appKey"];
///
/// @param appKey 设置的appKey
-(void)setAppKey:(NSString *)appKey;
/// 【必须】设置app 安全appSec
///
/// 使用例子:
/// [[CoraoolLib getInstance] setAppSec:@"appSec"];
///
/// @param appSec UserTracker :设置appSec
-(void)setAppSec:(NSString *)appSec;
Initialization example:
CoraoolLib *coraoolLib = [CoraoolLib getInstance];
[coraoolLib setAppId:@"your app id"];
[coraoolLib setAppKey:@"your appKey"];
[coraoolLib setAppSec:@"your appSec"];
let coraoolLib = CoraoolLib.getInstance()
coraoolLib?.setAppId("your app id")
coraoolLib?.setAppKey(""your appKey")
coraoolLib?.setAppSec("your appSec")
1.4 Other APIs
1.4.1 Login user ID
When users log in and log out, their login information needs to be updated in real time.
/// 【必须】设置app 的userId
///
/// 使用例子:
/// [[CoraoolLib getInstance] setUserId:@"1234"];
///
/// @param userId :设置app 的登录用户的userId
-(void)setUserId:(NSString *)userId;
Usage example:
/// 设置app 的userId
///
/// 使用例子:
/// [[CoraoolLib getInstance] setUserId:@"1234"];
///
/// @param userId :设置app 的登录用户的userId
-(void)setUserId:(NSString *)userId;
1.4.2 Log switch
The log will be opened during the development phase, and the release package will automatically close the log.
1.4.3 Strict mode
none
2. Buried interface
2.1 Tracking buried points
2.1.1 Buried point submission
Interface definition
@interface CoraoolUTBuilder : NSObject
/// UserTracker :发送日志
/// @param eventData 发送的数据,字典类型
-(void)track:(NSDictionary *)eventData;
Parameter Description:
parameter | explain | illustrate |
---|---|---|
eventData | Buried point data map | It is not recommended to manually construct buried point data constructed through the CoraoolUTBuilder subclass, as it is prone to errors. |
2.1.2 Control buried points
CoraoolLib *coraoolLib = [CoraoolLib getInstance];
CoraoolUTControlBuilder *builder = [[CoraoolUTControlBuilder alloc] initWithEventName:@"PAGE_MAIN"];
NSMutableDictionary *properties = [NSMutableDictionary new];
[properties setValue:@"业务参数value1" forKey:@"业务参数key1"];
[builder setProperties:properties];
[builder setProperty:@"updatePagePropKey1" value:@"updatePagePropVal1"];
[coraoolLib track:builder.build];
Field | type | Is it necessary | explain | illustrate |
---|---|---|---|---|
eventName | string | yes | event name | Identify where the user is on the page |
properties | Map<String, Object> | no | event parameters | Business parameters associated with the current event, used for offline analysis |
property_key | string | no | event parameter key | Business parameters associated with the current event, used for offline analysis |
property_value | string | no | event parameter value | Business parameters associated with the current event, used for offline analysis |
2.1.3 Exposure and buried points
CoraoolLib *coraoolLib = [CoraoolLib getInstance];
CoraoolUTExposeBuilder *builder = [[CoraoolUTExposeBuilder alloc] initWithEventName:@"PAGE_MAIN"];
NSMutableDictionary *properties = [NSMutableDictionary new];
[properties setValue:@"业务参数value1" forKey:@"业务参数key1"];
[builder setProperties:properties];
[builder setProperty:@"updatePagePropKey1" value:@"updatePagePropVal1"];
[coraoolLib track:builder.build];
Field | type | Is it necessary | explain | illustrate |
---|---|---|---|---|
eventName | string | yes | event name | Identify where the user is on the page |
properties | Map<String, Object> | no | event parameters | Business parameters associated with the current event, used for offline analysis |
property_key | string | no | event parameter key | Business parameters associated with the current event, used for offline analysis |
property_value | string | no | event parameter value | Business parameters associated with the current event, used for offline analysis |
2.1.4 Custom buried points
CoraoolLib *coraoolLib = [CoraoolLib getInstance];
CoraoolUTCustomBuilder *builder = [[CoraoolUTCustomBuilder alloc] initWithEventName:@"PAGE_MAIN"];
NSMutableDictionary *properties = [NSMutableDictionary new];
[properties setValue:@"业务参数value1" forKey:@"业务参数key1"];
[builder setProperties:properties];
[builder setProperty:@"updatePagePropKey1" value:@"updatePagePropVal1"];
[coraoolLib track:builder.build];
Field | type | Is it necessary | explain | illustrate |
---|---|---|---|---|
eventName | string | yes | event name | Identify where the user is on the page |
eventPage | string | yes | Event related page | Custom buried points must also specify associated page parameters. |
properties | Map<String, Object> | no | event parameters | Business parameters associated with the current event, used for offline analysis |
property_key | string | no | Business parameter key | Business parameters associated with the current event, used for offline analysis |
property_value | string | no | Business parameter value | Business parameters associated with the current event, used for offline analysis |
2.1.5 Page burying points
2.1.5.1 Manual buried points
Interface definition
1. Called when the page is displayed
/// UserTracker :页面埋点
/// page 埋点,trackPageStart 和trackPageEnd 成对出现,建议在viewDidAppear
/// 设计思路:
/// 在start的时候,启动以页面对象为key的数据
/// 在end的时候,会上传日志信息
///
/// 使用例子:
/// [[CoraoolLib getInstance] trackPageStart:self pageName:@"FirstViewController"];
///
/// @param pageObject 当前页面实例
/// @param pageName 页面名称
-(void)trackPageStart:(id)pageObject pageName:(NSString *)pageName;
2. 页面退出的时候调用
/**
UserTracker :页面埋点
page 埋点,start 和end 成对出现,建议在viewDidDisappear
*/
/// UserTracker :页面结束埋点,会传参数
///
/// 使用例子:
/// [[CoraoolLib getInstance] trackPageEnd:self];
///
/// @param pageObject 当前页面实例
-(void)trackPageEnd:(id)pageObject;
Parameter Description:
method name | parameter | explain | illustrate |
---|---|---|---|
trackPageStart | pageObject | Page Activity object | |
pageName | Custom page name | ||
trackPageEnd | pageObject | Page Activity object | |
pageName | Custom page name |
-(void)viewDidAppear:(BOOL)animated
{
[super viewDidAppear:animated];
[[CoraoolLib getInstance] trackPageStart:self pageName:@"FirstViewController"];
}
-(void)viewDidDisappear:(BOOL)animated
{
[super viewDidDisappear:animated];
[[CoraoolLib getInstance] trackPageEnd:self];
}
2.1.6 Get device id
get device id
Sample code
NSString *deviceId = [CoraoolLib deviceId];
字段 | Is it necessary | explain | illustrate | |
---|---|---|---|---|
result | string | yes | device id | get device id |
2.2 GTX burying protocol
none
3. Invoke API interface
Coraool SDK provides API-based data services and defines a set of universal and caller-friendly interfaces. Invoke interface calls initiated through Coraool SDK usually include the following four steps:
3.1 Construct Response
CoraoolResponse
It is an encapsulation of the Coraool API protocol and contains 3 fields. If you don’t care about the result of the request, you can directly use this class to receive the request result; if there is business data that needs to be processed, you need to inherit this class and add the result field. It also provides getter and setter methods. According to the Coraool API protocol, the business parameters returned by the API call will be stored in this Map structure. The SDK will automatically deserialize it to facilitate direct use by the application layer.
Parameter Description:
parameter | type | explain | illustrate |
---|---|---|---|
success | boolean | Is this request successful? | The returned result is valid if and only if this field is true |
code | int | error code | 200 means success, other values are similar to the definition of http status. If a negative number is returned, it means that an exception occurred in the SDK itself. The specific error code CoraoolResponse is defined by the constant |
message | string | Text description of the request result | It is only used to assist in troubleshooting the request process and cannot be used to judge business logic. It is always returned when the request is successful "SUCCESS" . If an error or exception occurs in the request, the corresponding error description information is returned, which can be used for troubleshooting or feedback to technical support; |
result | any | Custom business data types | If you need to pay attention to the result of the request, according to the Coraool API protocol, the data will be stored in a JSON object with result as the key. |
Parameter sample
#define SYSTEM_ERROR -2
#define REQUEST_ERROR -3
#define RESPONSE_ERROR -4
@interface CoraoolResponse : NSObject
@property bool success;
@property int code;
@property (strong,nonatomic)NSString *message;
@property long connectTimeout;
@property (strong,nonatomic)NSDictionary *result;
@end
3.2 Construct Request
Construct request parameters that conform to the Coraool API protocol by creating an object of CoraoolRequest type.
Parameter Description:
parameter | type | explain | illustrate |
---|---|---|---|
apiName | string | Interface name | Business interface registered on Coraool open API, for exampleopen.coraool.bingoplus.home.game.ranking |
apiVersion | string | Interface version | Business version registered on Coraool open API, e.g.1.0.0 |
data | any | Request parameters | Specific request parameter object |
connectTimeout | int | Link timeout in milliseconds | Network connection timed out |
readTimeout | int | Read timeout in milliseconds | Return data stream read timeout |
callbackOnMainThread | boolean | Callback to the main thread switch | Whether to call back to the UI main thread, the default is true. For data that is not directly displayed, it is recommended to set it to false, so that the request can be called back to the background thread to facilitate secondary processing of the data. |
Sample code:
CoraoolRequest *request = [CoraoolRequest new];
request.apiVersion = @"1.0.0";
request.apiName = @"open.coraool.bingoplus.home.game.ranking";
NSMutableDictionary *dict = [NSMutableDictionary new];
[dict setValue:@"登录用户ID" forKey:@"userId"];
[dict setValue:@"appsFlyer的ID" forKey:@"afId"];
[dict setValue:@"设备I" forKey:@"deviceId"];
[request setData:dict];
3.3 Initiate a request
The request API of the SDK is divided into synchronous requests and asynchronous requests, which are uniformly encapsulated under the interface class of CoraoolLib
3.3.1 Synchronous request
Interface definition:
/**
同步请求
*/
-(id )syncInvoke:(Class)modelClass
request:(CoraoolRequest *)request;
/**
同步请求
*/
-(void )syncInvoke:(Class)modelClass
request:(CoraoolRequest *)request
callback:(void (^)(CoraoolResponse *response))callback;
Parameter Description:
parameter name | type | explain | illustrate |
---|---|---|---|
clz | Class | Returns the Class of the object class | Class corresponding to the Response object class in the first step |
request | CoraoolRequest | Request parameters | Corresponding to the second step request parameter object |
callback | void (^)(CoraoolResponse ) | CallbackCallback | If the Callback interface parameter is provided, this request will always call back to a method in the Callback interface. For the definition of the interface method, see the next step. |
Return value description:
return value | type | explain | illustrate |
---|---|---|---|
response | CoraoolResponse | Return object class | The object instance corresponding to the first step Response |
Example description:
-(void)testSyncNetwork
{
CoraoolRequest *request = [CoraoolRequest new];
request.apiVersion = @"1.0.0";
request.apiName = @"open.coraool.bingoplus.home.game.ranking";
NSMutableDictionary *dict = [NSMutableDictionary new];
[dict setValue:@"123" forKey:@"userId"];
[dict setValue:@"afId" forKey:@"afId"];
[dict setValue:@"1234" forKey:@"deviceId"];
[request setData:dict];
request.connectTimeout = 5;
CoraoolLib *coraoolLib = [CoraoolLib getInstance];
CoraoolResponse *response = [coraoolLib syncInvoke:[CoraoolResponse class] request:request];
}
3.3.2 Asynchronous requests
Interface definition:
/// 异步请求
/// @param modelClass 返回后的类型
/// @param request 请求参数
/// @param success 成功后的block
/// @param failed 失败后的block
/// @param systemError 系统错误的block
-(void )asyncInvoke:(Class)modelClass
request:(CoraoolRequest *)request
success:(void (^)(CoraoolResponse *response))success
failed:(void (^)(CoraoolResponse *response))failed
systemError:(void (^)(CoraoolResponse *response))systemError;
Parameter Description:
parameter name | type | explain | illustrate | |
---|---|---|---|---|
clz | Class | Returns the Class of the object class | Class corresponding to the Response object class in the first step | |
request | CoraoolRequest | Request parameters | Corresponding to the second step request parameter object | |
success | response | Return object type | Returned when the request is processed correctly, corresponding to success=true | |
onFailed | code | int | Request error code | Corresponds to the scenario of success=false, if the signature is wrong, invalid token, etc. |
message | string | wrong description | ||
onSystemError | code | int | Request error code | Various system errors, such as network disconnection, authorization errors, data parsing exceptions, etc. |
message | string | wrong description |
Example description:
-(void)testAsyncNetwork
{
CoraoolRequest *request = [CoraoolRequest new];
request.apiVersion = @"1.0.0";
request.apiName = @"open.coraool.bingoplus.home.game.ranking";
NSMutableDictionary *dict = [NSMutableDictionary new];
[dict setValue:@"123" forKey:@"userId"];
[dict setValue:@"afId" forKey:@"afId"];
[dict setValue:@"1234" forKey:@"deviceId"];
[request setData:dict];
CoraoolLib *coraoolLib = [CoraoolLib getInstance];
[coraoolLib asyncInvoke:[NSDictionary class] request:request success:^(CoraoolResponse *response) {
NSLog(@"testAsyncNetwork success");
} failed:^(CoraoolResponse *response) {
NSLog(@"testAsyncNetwork failed");
} systemError:^(CoraoolResponse *response) {
NSLog(@"testAsyncNetwork systemError");
}];
}
4. Web access
4.1 Container layer access
4.1.1 Initializing the agent
example:
//Coraool 初始化 1:增加 WKScriptMessageHandler
//例子:
@interface WebViewController ()<WKScriptMessageHandler>
@end
4.1.2 Initializing the agent
- (void)viewDidLoad {
[super viewDidLoad];
//Coraool 初始化 2:增加 执行脚本
[[CoraoolLib getInstance] addJSBridge:self.wkWebView target:self];
}
4.1.3 Implementing agents
//Coraool 初始化 3:实现WKScriptMessageHandler
- (void)userContentController:(WKUserContentController *)userContentController didReceiveScriptMessage:(WKScriptMessage *)message
{
[[CoraoolLib getInstance] evaluateJS:self.wkWebView target:self userContentController:userContentController didReceiveScriptMessage:message];
}
4.1.4 Front-end call
example:
CoraoolJsLib.js example
let callbackId = 0;
let callbackMap = {};
class CoraoolJsLib{
static call(name, params, callback) {
//生成callbackId
let cbId = this.genCallbackId();
//添加到callbackMap
this.add(cbId, callback);
//组装方法和参数
let config = {name: name, params: params, callbackId: cbId};
let string = JSON.stringify(config);
window.webkit.messageHandlers.bridgeTest.postMessage(string);
}
//生成callbackId
static genCallbackId() {
return `Webview_callback_${callbackId++}`
}
//添加callback
static add(callbackId, callback) {
callbackMap[callbackId] = {
callback: callback
}
}
};
//注入全局方法,用于Native向h5回调
window.bridgeCallback = function(callbackId, res) {
let cb = callbackMap[callbackId];
let callback = cb["callback"];
if (callback) {
callback(res);
}
delete callbackMap.callbackId
}
js_bridge.html example
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Apache ECharts Demo</title>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/echarts.min.js"></script>
<style>
.event-button {
margin-right: 8px;
background-color: green;
color: white;
}
.page-button {
margin-right: 8px;
background-color: blue;
color: white;
}
.verify-button {
margin-right: 8px;
background-color: red;
color: white;
}
.dialog-button {
margin-right: 8px;
background-color: orange;
color: white;
}
</style>
</head>
<body>
<script type="text/javascript" src="./CoraoolJsLib.js"></script>
<div id="chart" style="width: 350px; height: 200px;"></div>
<script>
var chart = echarts.init(document.getElementById('chart'));
var option = {
xAxis: {
5. Abtest Interface
5.1 Initialize Abtest Function
The Abtest function can be enabled by overloading the isEnableAbtest method of the com.coraool.IParamProvider interface. Things to note:
- The function of the Abtest module is turned off by default and needs to be explicitly turned on before it can be used;
- The user identity will be used for the calculation of experimental bucketing, so parameters like userId need to be set as early as possible. It is recommended to complete it before calling the initialize method.
public class CApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
// Please set the user identity before SDK initialization, because it will be used in the subsequent initialization process.
CoraoolLib.getInstance().setUserId("123abc");
CoraoolLib.getInstance().setCustomDeviceId("my_unique_device_id");
CoraoolLib.getInstance().initialize(this, new IParamProvider() {
...
// By overloading this method, start the Abtest module function
@Override
public boolean isEnableAbtest() {
return true;
}
});
}
}
5.2 Get single experiment bucket
Interface definition
-(NSString *)getABTest:(NSString *)experimentName;
Parameter description
parameter name | explain | illustrate |
---|---|---|
experimentName | Experiment name | The name of the experiment created on the experiment platform |
Sample code
let bucket = CoraoolLib.getInstance().getABTest("FEEDS_OLD_USER_BASE");
5.3 Get a single experiment bucket object
Interface definition
-(CoraoolABTestModel *)getABTestObject:(NSString *)experimentName;
Parameter description
parameter name | explain | illustrate |
---|---|---|
experimentName | Experiment name | The name of the experiment created on the experiment platform |
Sample code
let model = CoraoolLib.getInstance().getABTestObject("FEEDS_OLD_USER_BASE")
5.4 Get all experimental buckets
Interface definition
-(NSArray<CoraoolABTestModel*> *)getABTestListAll;
Parameter description
parameter name | explain | illustrate |
---|---|---|
none | none | none |
Sample code
let models = CoraoolLib.getInstance().getABTestListAll()
Comments (0)